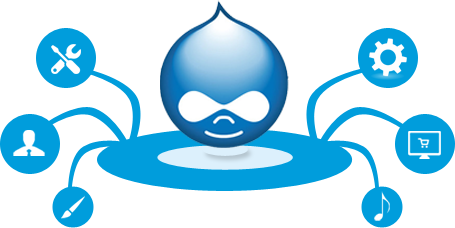
Drupal 7 开发指引
常用命令及代码
开发环境
# 确认命令行环境和 web 环境为同一套 php 环境
php -r 'echo get_include_path()."\n";'
pear config-get php_dir
pear install PHP_CodeSniffer-2.9.1 # 安装 PHP 语法检查器
# 最新的 3.4.2 也能和 coder 一起工作
pear install PHP_CodeSniffer
downloading PHP_CodeSniffer-3.4.2.tgz ...
Starting to download PHP_CodeSniffer-3.4.2.tgz (637,880 bytes)
...........................................done: 637,880 bytes
install ok: channel://pear.php.net/PHP_CodeSniffer-3.4.2
drush dis -y captcha, googleanalytics
drush en -y views_ui, field_ui, og_ui, devel, coder_review, variable_admin
drush vset video_ffmpeg_path /usr/local/bin/ffmpeg
drush vset preprocess_js 0
drush vset preprocess_css 0
drush cc all
Entity 处理
# 按 ID 查 entity 详情
$node = entity_load_single('node', $nid);
dpm($node); # 调试变量,需要打开 Devel 模块。
dpm(entity_extract_ids('node', $node)); # 获取 Node ID,版本 ID,Bundle 名
https://www.drupal.org/docs/7/creating-custom-modules/howtos/how-to-use-entityfieldquery-for-drupal-7
JS 模版
/**
* @file
*/
/* jshint esversion: 6 */
/* jshint unused:false, strict: false */
/* globals Drupal:object, jQuery:object */
(function ($) {
Drupal.behaviors.<MODULE_NAME> = {
/**
* Attach behaviors to enhance UIs.
*
* @param context document
* @param settings Drupal.settings
*
* @see Drupal.attachBehaviors JSDoc.
*/
attach: function(context, settings) {
$('body', context).once('-body-attach', function () {
// once finished.
});
}
};
})(jQuery);
PHP 与 JS 传值
drupal_add_js(array('<MODULE_NAME>' => $settings_array), 'setting');
$link = l('LINK_NAME', 'PATH_OR_URL', array(
'attributes' => array(
'class' => array('button'),
'target' => '_blank',
)
));
调试相关 settings.php 设置
$conf['theme_debug'] = TRUE;
$conf['https'] = TRUE;
$conf['drupal_http_request_fails'] = FALSE;
$conf['error_level'] = ERROR_REPORTING_DISPLAY_ALL;
#$conf['error_level'] = ERROR_REPORTING_HIDE;
Drush 数据库运维
# Backup site status
drush sql-dump | bzip2 -c > PATH_OF_BZ2_DUMP
# Restore site status
redis-cli FLUSHALL
drush sql-drop -y
bzip2 -dc PATH_OF_BZ2_DUMP | `drush sqlc`
MySQL 数据巡查
# 90 天内活跃(登录)用户数
SELECT count(uid)
FROM users
WHERE status = 1
AND from_unixtime(login) BETWEEN DATE_SUB(NOW(), INTERVAL 90 DAY) AND NOW();
常用资料
编码规约
Form 生命周期图
参考链接
数据库相关
巡查 SQL
查看 Drupal DB API 生成的 SQL 语句:
$sql = $query->__toString();
故障排除
Drush 连不上 Drupal 网站
正确运行 Drush 命令,需要相同命令行环境下 mysql 命令行客户端可以连上 Drupal 网站的数据库。默认 MAMP 环境下,单独安装的 mysql 命令行因为连不上 MySQL 数据库,会产生如下错误:
Command pm-updatestatus needs a higher bootstrap level to run - you will need to invoke drush from a more functional Drupal environment to run this command. [error]
The drush command 'ups' could not be executed. [error]
Drush was not able to start (bootstrap) the Drupal database. [error]
Hint: This may occur when Drush is trying to:
* bootstrap a site that has not been installed or does not have a configured database. In this case you can select another site with a working database setup by specifying the URI to use with the --uri parameter on the command line. See `drush topic docs-aliases` for details.
* connect the database through a socket. The socket file may be wrong or the php-cli may have no access to it in a jailed shell. See http://drupal.org/node/1428638 for details.
Drush was attempting to connect to:
Drupal version : 7.59
Site URI :
Database driver : mysql
Database hostname : 127.0.0.1
Database port : 3306
Database username : root
Database name : default
PHP configuration : /Applications/MAMP/bin/php/php7.0.26/conf/php.ini
PHP OS : Darwin
Drush script : /usr/local/bin/drush
Drush version : 8.1.14
Drush temp directory : /tmp
Drush configuration :
Drush alias files :
Drupal root : /var/www/html
Drupal Settings File : sites/default/settings.php
Site path : sites/default
通过在 ~/.profile 中,把 MAMP 的 php 和 mysql 命令行客户端所在目录的路径加入到 PATH 环境变量,可以解决此类问题。
export PATH=/Applications/MAMP/Library/bin:/Applications/MAMP/bin/php/php7.0.27/bin:$PATH
环境配置
安装指定版本 PHP
# 安装
sudo apt install php7.1-cli php7.1-fpm php7.1-json php7.1-common php7.1-mysql php7.1-zip php7.1-gd php7.1-mbstring php7.1-curl php7.1-xml php7.1-bcmath php7.1-json php-pear
sudo apt install php7.3-cli php7.3-fpm php7.3-json php7.3-common php7.3-mysql php7.3-zip php7.3-gd php7.3-mbstring php7.3-curl php7.3-xml php7.3-bcmath php7.3-json php-pear
sudo pear install PHP_CodeSniffer
# Drush
# 访问 https://github.com/drush-ops/drush/releases 下载 drush.phar
Nginx 设置
CRON
# 每小时执行
# Mac
0 * * * * /usr/local/bin/wget -O - -q -t 1 CRON_URL
函数调试环境设置
<?php
/**
* @file
* The debug file of Drupal functions.
*/
/**
* Root directory of Drupal installation.
*/
define('DRUPAL_ROOT', getcwd());
$_SERVER['SERVER_NAME'] = 'dev.moha.online';
$_SERVER['HTTP_HOST'] = 'dev.moha.online';
/**
* Required core files needed to run PHP script.
*/
require_once DRUPAL_ROOT . '/includes/bootstrap.inc';
require_once DRUPAL_ROOT . '/includes/common.inc';
require_once DRUPAL_ROOT . '/includes/module.inc';
require_once DRUPAL_ROOT . '/includes/unicode.inc';
require_once DRUPAL_ROOT . '/includes/path.inc';
require_once DRUPAL_ROOT . '/includes/file.inc';
// Bootstrap Drupal to at least the database level so it can be accessed.
drupal_bootstrap(DRUPAL_BOOTSTRAP_VARIABLES);